How to Create a Python Calculator
- siddhant rana
- Jul 1, 2023
- 2 min read
Python is a powerful programming language that can be used to create a variety of applications, including calculators. In this blog post, we will show you how to create a simple calculator in Python.
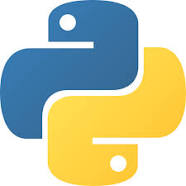
Prerequisites
Before you start, you will need to have a basic understanding of Python. If you are new to Python, I recommend checking out the Python tutorial: https://docs.python.org/3/tutorial/.
Step 1: Create a file for the calculator
The first step is to create a file for the calculator. You can name the file whatever you want, but I recommend calling it calculator.py.
Step 2: Prompt for user input
The next step is to prompt the user for input. You will need to ask the user for two numbers, as well as the operation they want to perform.
Python first_number = input("Enter the first number: ") second_number = input("Enter the second number: ") operation = input("Enter the operation: ") Use code with caution. Learn more content_copy
Step 3: Perform the operation
Once you have the user's input, you can perform the operation. The following code shows how to perform addition, subtraction, multiplication, and division:
Python if operation == "+" : result = first_number + second_number elif operation == "-" : result = first_number - second_number elif operation == "*" : result = first_number * second_number elif operation == "/" : result = first_number / second_number Use code with caution. Learn more content_copy
Step 4: Display the result
Finally, you need to display the result to the user. You can do this using the print() statement:
Python print(f"The result is {result}") Use code with caution. Learn more content_copy
Step 5: Save the file and run the program
Once you have completed all of the steps, you need to save the file and run the program. You can do this by opening a terminal window and navigating to the directory where you saved the file. Then, you can run the program by typing the following command:
Code snippet python calculator.py
Comments